• Where?
• Language
• Events
• Commands
• Properties
• Settings
• Where?^
With Music Studio, you can control the instruments’ behavior and make them more flexible and intelligent by adding some scripts.
Each mode of each Instrument can have a separate Script:
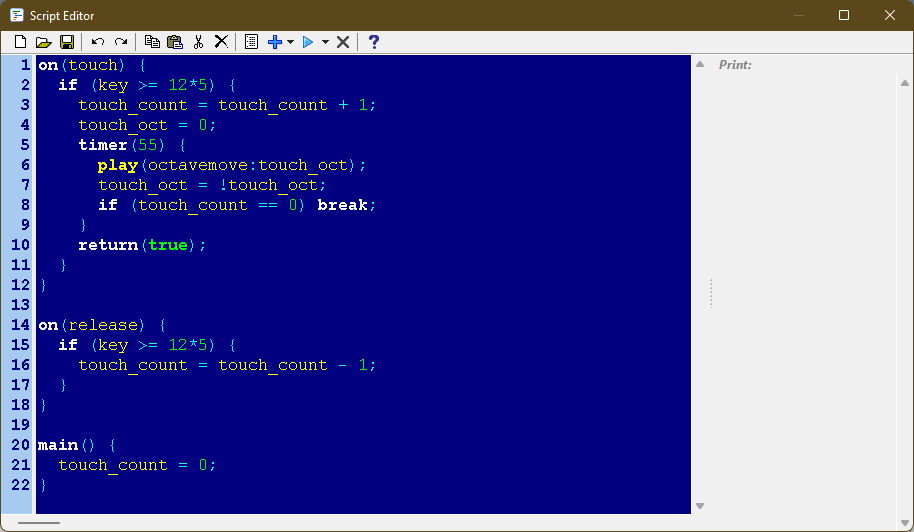
• Language^
Main: Runs one time at the start.
Main() {
print("Hello, World!");
}
Hello, World!
Main() {
message("Hello, World!");
}
Main() {
a = 2; // Integer
b = 1.45; // Float
c = 3, 5, 2.6, 4; // Array, for example c[3] is 4
print("Sum:", a + b + c[3]);
}
Sum: 7.45
MyFunc() {
return(10);
}
Main() {
print("MyFunc: " + MyFunc());
}
MyFunc: 10
MyFunc(a, b) {
return(a + b);
}
Main() {
print("MyFunc: " + MyFunc(a:1, b:2));
}
MyFunc: 3
MyFunc(a, b:5) { // b has a default value
return(a + b);
}
Main() {
print("MyFunc: " + MyFunc(a:1));
}
MyFunc: 6
Main() {
a = 3 - 1;
if (a == 2)
print("2");
else
print("It is not 2");
}
2
Main() {
a = 3 - 1;
switch (a) {
case 1: print("1"); break;
case 2, 3: print("2 or 3"); break;
case 4..7: print("4, 5, 6 or 7"); break;
case 8, 12..14: print("8, 12, 13 or 14"); break;
default: print("something else"); break;
}
}
2 or 3
Main() {
for (i: 1..3)
print(i);
}
1
2
3
Main() {
for (i: 1..5 @ 2)
print(i);
}
1
3
5
Main() {
i = 1;
while (i <= 5) {
print(i);
i = i + 2;
}
}
1
3
5
Main() {
i = 1;
while (true) {
print(i);
i = i + 2;
if (i > 5) break;
}
}
1
3
5
Main() {
i = 1;
timer (100) { // each 100 milliseconds
print(i);
i = i + 2;
if (i > 5) break;
}
}
1
3
5
Main() {
print(Min(5, 3, 8, 1));
print(Max(5, 3, 8, 1));
print(MinMax(5, 1, 4));
print(pi);
print(Random); // between 0 and 1
print(Random(10)); // between 0 and 9
print(time); // Current Time in milliseconds
}
1
8
4
3.14159265358979
0.0476205942686647
6
3865061865243
Also byte, shortint, word, integer, longint, abs, sqrt, sqr, sin, cos, arctan, arcsin, arccos, ln, exp, fact, int, and not
• Events^
on (touch): A clavier is touched (on the app or MIDI keyboard)
available data:
sound: Sound index
id: Play ID
key: Clavier number, between 0 and 127
velocity: Touch velocity, between 0 and 127
pedal: Pedal state, 0 or 1
on (aftertouch): A clavier is pressed after touching (on a MIDI device with aftertouch support)
available data:
sound: Sound index
id: Play ID
key: Clavier number, -1 or between 0 and 127 (-1 for Channel Aftertouch)
velocity: Pressure, between 0 and 127
pedal: Pedal state, 0 or 1
on (release): A clavier is released (on the app or MIDI keyboard)
available data:
sound: Sound index
id: Play ID
key: Clavier number, -1 or between 0 and 127 (-1 for Channel Aftertouch)
velocity: Release velocity, between 0 and 127 (if supported)
pedal: Pedal state, 0 or 1
on (pitchbend): Pitch-bend wheel or joystick (horizontally) is moved (on the app or MIDI)
available data:
level: between -8192 and 8192
on (modulate): Mod wheel or joystick (vertically) is moved (on the app or MIDI)
available data:
level: between -127 and 127
on (pedalon): Pedal is pressed (on the app or MIDI)
on (pedaloff): Pedal is released (on the app or MIDI)
on (control): A control is touched or changed (on a MIDI device)
on (program): Program is changed (on a MIDI device)
on (mode): A DNC button is touched (on the app)
on(touch) {
if (key >= 60 && velocity >= 90) print(key, velocity);
return(false); // return(true) if you have done all you need
}
on(aftertouch) {
effect[2].enable = velocity != 0; // effect[2] is pitch-shake
effect[2].Depth = velocity;
effect[2].Speed = 30;
}
• Commands^
Play (sound:, id:, key:, velocity:, balance:, volume:, pan:, keymove:, octavemove:, tune:, pitchmove:, pedal:, attack:, decay:, sustain:, release:, autorelease:, connectkey:, connectspeed:)
Plays a Music note
on(touch) {
if (velocity >= 100) play(sound:2); else play(sound:0);
return(true);
}
Stop (id:, cut)
Stops a playing note, use cut to stop immediately
on(release) {
stop(cut); // stop all playing notes immediately
return(true);
}
StopAll (cut)
Stops all playing notes
Change (velocity:, balance:, pitchmove:)
Changes current playing notes
Playing
Count the playing notes
KeyToPitch (key:)
Calculates the pitchmove value from the key number, the key can be a float value
Exit (mode:)
Changes DNC mode
on(release) {
Exit; // Activate the Default mode
}
on(release) {
Exit(2); // Activate the DNC mode 2
}
• Properties^
keyboard.count: Keyboard key count (Read only)
keyboard.start: Keyboard first key (Read only)
keyboard.chord: Chord key count (Read only)
keyboard.chordscanlower: Lower Chord Scan button state (Read only)
keyboard.chordscanupper: Upper Chord Scan button state (Read only)
keyboard.chordmemory: Chord Memory button state (Read only)
keyboard.autofill: Upper Auto Fill button state (Read only)
keyboard.synchrostart: Synchro Start button state (Read only)
keyboard.synchrostop: Synchro Stop button state (Read only)
fx.Count: Count of added FXs in track (Read only)
fx.Enable: Is track FXs enabled (Read/Write)
fx.EnabledCount: Count of enabled FXs in track (Read only)
fx.Volume: Track Volume (Read/Write)
fx.Balance: Track Balance (Read/Write)
fx.Tune: Track Tune value (Read/Write)
fx.Keymove: Track transpose (Read/Write)
fx.Octavemove: Track move octave (Read/Write)
fx.Stabilitypercent: Track stability percent (Read/Write)
fx.ForceLoop: Track Force Loop (Read/Write)
fx[Index].Enable: Is an FX enabled? (Read/Write)
Change Effects parameters: (Read/Write)
Index is: 0 = Repeat, 1 = Mono/Legato, 2 = Pitch Shake, and 3 = Volume/Pan Shake
effect[Index].Enable
effect[Index].StartDelay
effect[Index].ByPedal
effect[Index].StopByRelease
effect[Index].Mode
effect[Index].Speed
effect[Index].Depth
effect[Index].Gain
on(modulate) {
effect[2].enable = level != 0;
effect[2].Depth = abs(level);
if (level > 0)
effect[2].Speed = 20;
else
effect[2].Speed = 40;
}
• Settings^
You can let the user set some variables of scripts on a settings page.
setting(type, name:, title:, reset:, from:, to:);
The type can be one of text, slider, or toggle
on(aftertouch) {
effect[2].enable = velocity != 0;
effect[2].Depth = (velocity * aftertouch_depth) / 5;
effect[2].Speed = 5 * aftertouch_speed;
}
on(modulate) {
effect[2].enable = level != 0;
effect[2].Depth = (abs(level)*modulate_depth) / 5;
if (level > 0)
effect[2].Speed = 4*modulate_speed;
else
effect[2].Speed = 8*modulate_speed;
}
main() {
setting(text, title:"Aftertouch");
setting(slider, name:"aftertouch_speed",
title:"Speed", reset:5, from:1, to:20);
setting(slider, name:"aftertouch_depth",
title:"Depth", reset:5, from:1, to:20);
setting(text, title:"Modulation");
setting(slider, name:"modulate_speed",
title:"Speed", reset:5, from:1, to:20);
setting(slider, name:"modulate_depth",
title:"Depth", reset:5, from:1, to:20);
}